Learn from the basics about JSON data. We will create JSON data, add element, save it to a JSON file and view json on a Firefox browser. I’m currently using Windows 10 and writing the codes on Microsoft Studio Code app.
Create a JSON data:
import json data = {} data['name'] = 'Aprilyn' data['gender'] = 'female' print(data) Result: {'name': 'Aprilyn', 'gender': 'female'}
Adding element on JSON:
data.update({'age':21}) print(data) {'name': 'Aprilyn', 'gender': 'female', 'age': 21}
Saving data to Json file:
import json data = {} data['name'] = 'Aprilyn' data['gender'] = 'female' data['age'] = 21 filename = 'd:/json_sample_data.json' with open(filename, 'w') as outfile: json.dump(data, outfile)
This code will save data on drive D of my computer. You can open the json using your internet browser like Firefox. You can see something like this:
{'name': 'Aprilyn', 'gender': 'female', 'age': 21}
This is a raw view of json. If you want to see it in Json format, we have to install addons on firefox. Please open this link using firefox: Jsonview addons
This is a raw view of JSON. If you want to see it in JSONformat, we have to install add-ons on Firefox. Please open this link using Firefox browser:
View JSON in a reading format
Activate the add-ons and restart your Firefox by closing and reopening it again. Then open the json file again and you should see something like this.
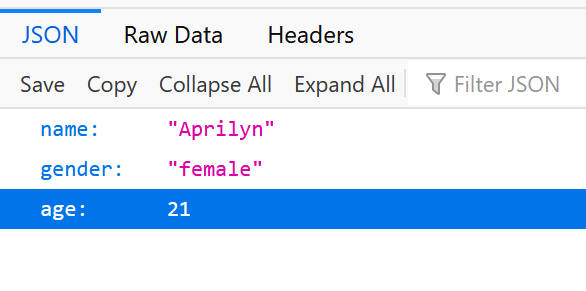
Now, I put all the codes together:
import json def create_data(): data = {} data['name'] = 'Aprilyn' data['gender'] = 'female' #data['age'] = 21 data.update({'age':21}) print(data) return data def save_json(data) filename = 'd:/json_sample_data.json' with open(filename, 'w') as outfile: json.dump(data, outfile) if __name__ == "__main__": data = create_data() save_json(data)